When considering web links as sources and targets, network analysis algorithms have several practical applications across various fields. Here are some key uses:
Web Page Ranking and Search Engine Optimization (SEO)
- PageRank Algorithm: This algorithm, developed by Google, uses the network of web links to rank web pages based on their importance. Pages with more incoming links from high-ranking pages are considered more important and are ranked higher in search results.
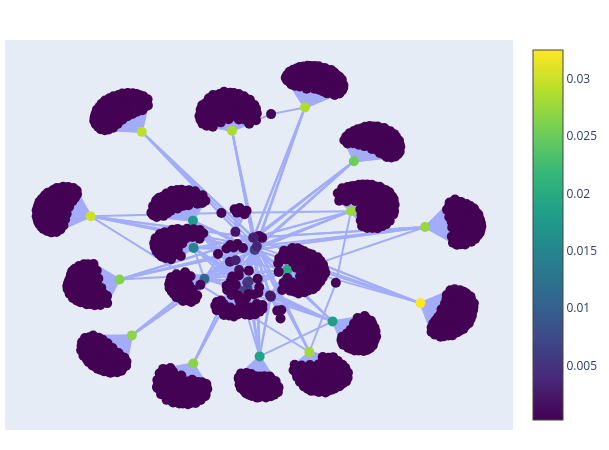
Spam Detection and Fraud Prevention
- Link Spam Analysis: Network analysis can help identify link spam farms, which artificially inflate a page’s ranking by creating numerous links from low-quality sites. Algorithms can detect anomalies in link patterns to flag and mitigate such activities.
Network Structure and Community Detection
- Community Detection: By analyzing the network of web links, you can identify clusters or communities of web pages that are highly interconnected. This helps in understanding the topical structure of the web and can be useful for personalized search results and content recommendation.
Centrality and Influence Analysis
- Centrality Measures: Calculate degree centrality, betweenness centrality, and closeness centrality to identify key web pages (nodes) in the network. For example, a page with high degree centrality has many links pointing to it, indicating its importance or influence.
Information Spread and Virality
- Information Diffusion: Analyze how information spreads across the web by studying the network of links. This can help in understanding which web pages are most effective at disseminating information and which paths information is likely to take.
Web Graph Visualization and Exploration
- Interactive Visualization: Use tools like those provided by the Network Repository (NR) or Tom Sawyer Perspectives to visualize the web link network. This helps in exploring the structure of the web, identifying key nodes, and understanding the relationships between web pages in real-time.
Anomaly Detection and Security
- Anomaly Detection: Network analysis can help detect unusual patterns in web link data, such as sudden spikes in links to a particular page, which could indicate malicious activity like phishing or malware distribution.
Personalization and Filtering
- Personalized Search: Use network analysis to adjust ranking scores based on user preferences or specific topics of interest. This can improve the relevance of search results and enhance the user experience.
Dynamic Network Analysis
- Temporal Analysis: Analyze how the network of web links changes over time. This can help in understanding trends, identifying emerging topics, and predicting future changes in the web landscape.
By applying these network analysis algorithms, you can gain valuable insights into the structure and dynamics of the web, which can be used to improve search engines, detect spam and fraud, and enhance user experiences.
Information Dissemination Via Network Analysis
To understand which web pages are most effective at disseminating information and the paths information is likely to take, several algorithms from network analysis can be employed. Here are some key algorithms and how you can implement them using Python with the NetworkX library.
Centrality Measures
Centrality measures are crucial for identifying the most influential nodes (web pages) in a network.
Degree Centrality
This measures the number of edges connected to a node, indicating its popularity or connectivity.
Betweenness Centrality
This measures the proportion of shortest paths between all pairs of nodes that pass through a given node, indicating its role as a bridge or intermediary.
Closeness Centrality
This measures the average shortest path length from a node to all other nodes, indicating how quickly information can spread from that node.
Information Diffusion Models
Models like the Independent Cascade Model can simulate how information spreads through a network.
Here is an example of how you can use NetworkX to calculate centrality measures and simulate information diffusion:
import networkx as nx
import numpy as np
# Create a sample network
G = nx.DiGraph()
G.add_edges_from([
('A', 'B'), ('A', 'C'), ('B', 'D'), ('C', 'D'), ('D', 'E'),
('E', 'F'), ('F', 'A'), ('F', 'B')
])
# Calculate centrality measures
degree_centrality = nx.degree_centrality(G)
betweenness_centrality = nx.betweenness_centrality(G)
closeness_centrality = nx.closeness_centrality(G)
print("Degree Centrality:", degree_centrality)
print("Betweenness Centrality:", betweenness_centrality)
print("Closeness Centrality:", closeness_centrality)
# Independent Cascade Model simulation
def independent_cascade(G, seed_nodes, influence_probability):
# Initialize the set of active nodes
active_nodes = set(seed_nodes)
# Initialize the set of newly activated nodes
new_active_nodes = set(seed_nodes)
while new_active_nodes:
# Update the set of newly activated nodes
new_active_nodes = set()
for node in new_active_nodes:
for neighbor in G.neighbors(node):
if neighbor not in active_nodes and np.random.rand() < influence_probability:
new_active_nodes.add(neighbor)
active_nodes.add(neighbor)
return active_nodes
# Example usage of Independent Cascade Model
seed_nodes = ['A']
influence_probability = 0.5
activated_nodes = independent_cascade(G, seed_nodes, influence_probability)
print("Activated Nodes:", activated_nodes)
Explanation
- Centrality Measures: The code calculates degree, betweenness, and closeness centrality for each node in the network. These measures help identify the most influential nodes in terms of connectivity, bridging, and information spread.
- Independent Cascade Model: This model simulates how information spreads from a set of seed nodes through the network. The
independent_cascade
function takes the graphG
, a list of seed nodes, and an influence probability as inputs and returns the set of nodes that become active (i.e., receive the information).
This example demonstrates how to use NetworkX to analyze the structure of a web link network and simulate information diffusion, helping to understand which web pages are most effective at disseminating information and the paths information is likely to take.
Workflows in Network Analysis
In network analysis, different centrality measures can be used to create various workflows depending on the goals and context of the analysis. Here are some common centrality measures and the workflows they can be part of:
Degree Centrality
Definition: Degree centrality measures the number of direct connections (edges) a node has with other nodes in the network.
Workflows:
- Identifying Highly Connected Nodes: Use degree centrality to find nodes that are highly connected, such as influential individuals in a social network or key hubs in a transportation network.
- Spreading Information: Nodes with high degree centrality can quickly disseminate information to a large portion of the network, making them ideal for marketing or communication strategies.
- Network Robustness: Analyze the degree distribution to understand the resilience of the network to node failures or attacks.
Betweenness Centrality
Definition: Betweenness centrality measures the number of times a node lies on the shortest path between other nodes, indicating its role as a bridge or connector.
Workflows:
- Identifying Key Intermediaries: Use betweenness centrality to find nodes that control the flow of information or resources between different parts of the network. This is crucial in understanding communication dynamics or identifying potential bottlenecks.
- Network Optimization: Identify and remove or reinforce nodes with high betweenness centrality to optimize network flow and reduce dependencies on single nodes.
- Community Detection: Betweenness centrality can help in identifying nodes that connect different communities within a network.
Closeness Centrality
Definition: Closeness centrality measures the average length of the shortest paths from a node to all other nodes in the network, indicating how quickly a node can interact with all other nodes.
Workflows:
- Rapid Information Dissemination: Use closeness centrality to identify nodes that can quickly spread information or resources across the entire network, making them ideal for emergency response or broadcast scenarios.
- Central Nodes in Clusters: Closeness centrality is useful for finding central nodes within specific clusters or sub-networks.
- Network Efficiency: Analyze closeness centrality to understand the overall efficiency of the network in terms of communication and resource allocation.
Eigenvector Centrality
Definition: Eigenvector centrality measures a node’s influence based on the number and quality of its connections, considering the centrality of its neighbors.
Workflows:
- Identifying Influential Nodes: Use eigenvector centrality to find nodes that are connected to other highly influential nodes, which can help in understanding indirect influence and network behavior.
- Social Network Analysis: This measure is particularly useful in social networks to identify individuals who have significant influence through their associations with other key players.
- Malware Propagation: Eigenvector centrality can help in understanding how malware or information spreads through a network by identifying central nodes that are connected to other influential nodes.
PageRank Centrality
Definition: PageRank is a variant of eigenvector centrality that takes into account the direction and weight of links, originally developed for ranking web pages.
Workflows:
- Authority and Influence: Use PageRank to identify nodes that have authority and influence within the network, considering the direction and weight of connections.
- Citation Analysis: PageRank is useful in citation networks to determine the importance of documents or authors based on the quality and quantity of citations.
- Recommendation Systems: This measure can be used in recommendation systems to rank items based on their connectivity and the connectivity of their neighbors.
Using NetworkX
NetworkX is a Python library for the creation, manipulation, and study of the structure, dynamics, and functions of complex networks. Here’s how you can use NetworkX to calculate these centrality measures:
import networkx as nx
# Create a sample graph
G = nx.Graph()
G.add_edges_from([(1, 2), (1, 3), (2, 3), (2, 4), (3, 4), (4, 5)])
# Calculate degree centrality
degree_centrality = nx.degree_centrality(G)
print(degree_centrality)
# Calculate betweenness centrality
betweenness_centrality = nx.betweenness_centrality(G)
print(betweenness_centrality)
# Calculate closeness centrality
closeness_centrality = nx.closeness_centrality(G)
print(closeness_centrality)
# Calculate eigenvector centrality
eigenvector_centrality = nx.eigenvector_centrality(G)
print(eigenvector_centrality)
# Calculate PageRank centrality
pagerank_centrality = nx.pagerank(G)
print(pagerank_centrality)
By leveraging these centrality measures and the capabilities of NetworkX, you can create comprehensive workflows to analyze and understand the structure and dynamics of various types of networks.