Docker is one of the revolutionary technologies that has pushed the boundaries of software development since it inception. In this tutorial we will be learning the most important and commonly used docker commands using the 80/20 rule also popularly called the Pareto Principle.
The Pareto Principle or 80/20 rules simple states that 80 percent of results are the product of 20 percent of the activities. 20 percent of the most important activity produces the remaining 80 results. This principle can be seen in many areas of life. For example most people use 20% of the features of software most of the time. Thus 80% of our time we are using just 20% of the features of a particular tool.
We will be applying this same principle in learning docker. In other words when learning any tool or technology it is more efficient and effective for a person to know the most important and commonly used features that get 80% of the work done.
So we will pick the top 20% docker commands and tips that an individual will use most of the time in his software development journey.
First of all if you do not have docker installed on your system you can check out this link.
By the end of this post, you will learn
- what we mean by pareto principle and how to apply it to learning any technology
- top docker commands
- how to copy files to your docker container
- etc
To make our learning simple we will divide all the commands into sections. These sections include
- Commands For Gathering Docker Information
- Commands For Starting,Stopping and Running Docker
- Commands For Building and Destroying Docker
- Commands For Connecting to Docker
Docker Information Gathering
In this section we will explore the commands needed to gather information about docker images and docker containers
How to Get Docker Version Info
docker version
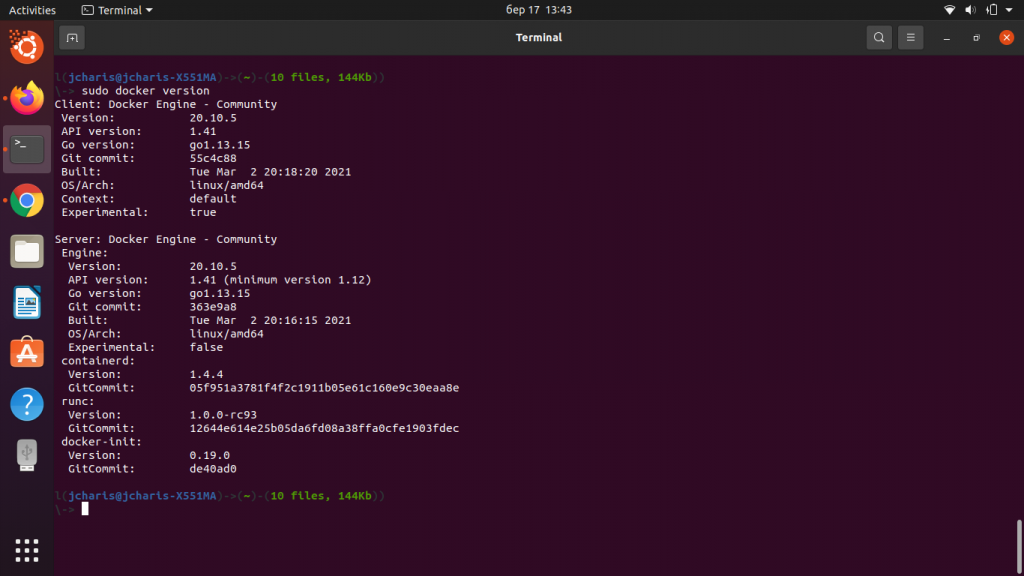
How to know how many docker images,containers and process you have
docker info
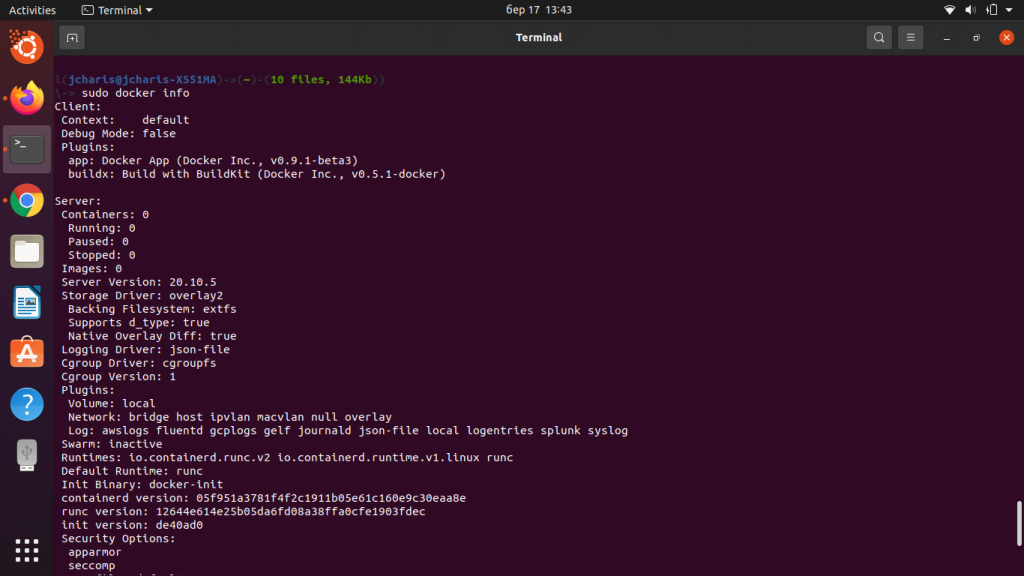
How to Search for A Docker Image
docker search alpine docker search jupyter
This command searches dockerhub for images that others have already builts and uploaded to the dockerhub repository
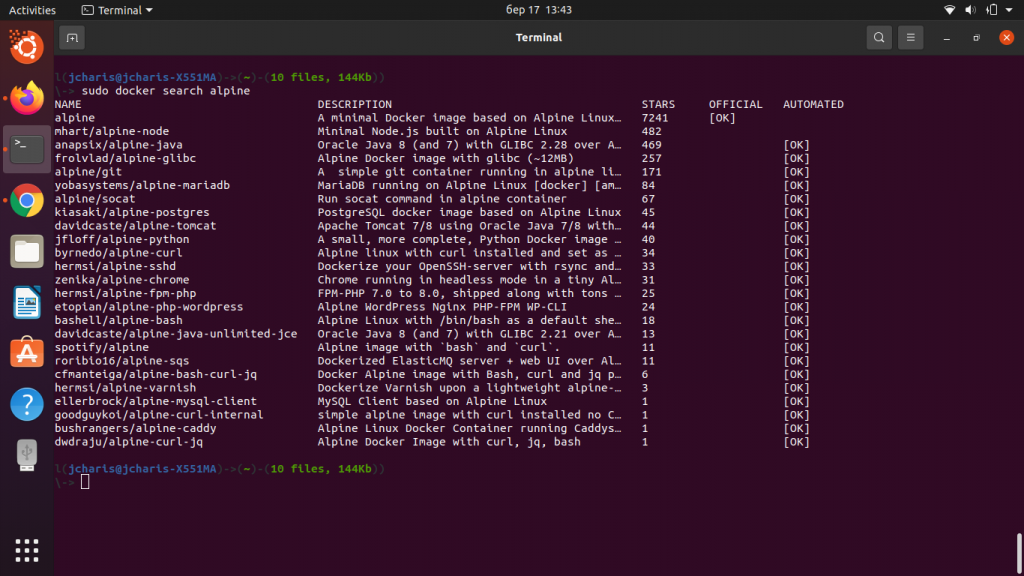
How to know what Docker Images you have
docker images
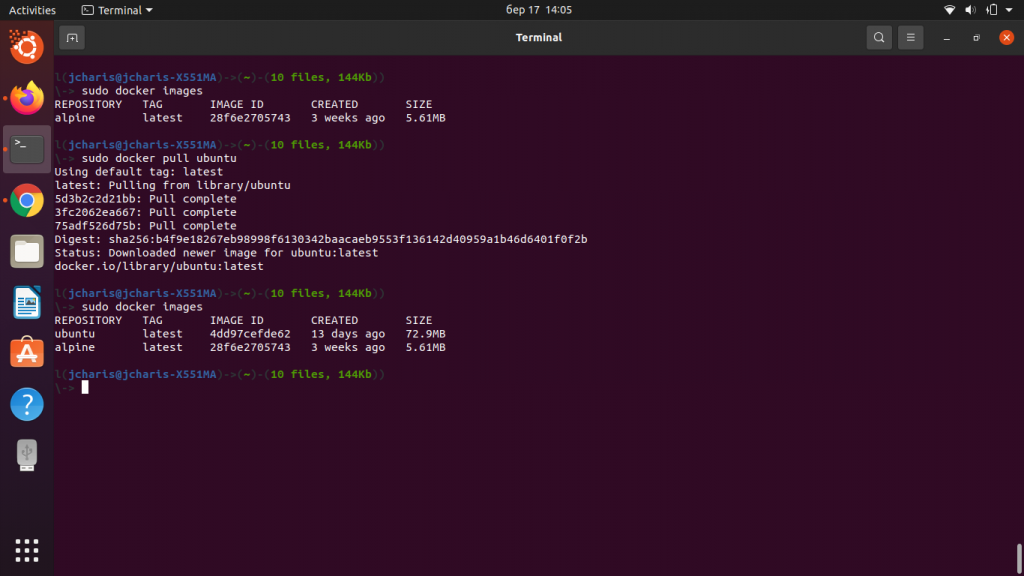
How to Download Docker Images (Docker Pull)
This will pull or download the specified docker image to your docker workspace. In our case we can pull the alpine docker image and the ubuntu docker image (OS)
docker pull alpine docker pull alpine:latest docker pull ubuntu
How to Get History of a Docker Image
You can also get the complete history of a docker image on your system using the history command
docker history alpine
Run,Start and Stop Docker Containers
After pulling or downloading a docker image which is like a mini- operating system on your local computer you can create containers – which is like an environment built from your docker image where you can package your applications and box your code to run it easily.
How to Run A Docker Container
docker run -p <LOCALHOST_PORT>:<CONTAINER_PORT> <DOCKER_IMAGE> docker run -p 8080:80 nginx
This will first of all create a container instance using the docker image you specify and spin it up. The -p specifies the port you will want to listen to. So in our case the 8080 is your localhost port and the 80 is the port from your docker instance that your localhost or your browser will be listening from.
You can also detach the running docker container from your current shell using the -d argument
docker run -p 8080:80 nginx -d
How to Run A Docker Container with a Custom Container Name
docker run --name mycontainer_one - p 8080:80 -d nginx
How to Run a Docker Container in an Interactive Mode
You can directly run a docker container and connect to it using the interactive mode -it. This allows you to ‘ssh’ into your docker container instance.
docker run --name mycontainer_one -it ubuntu:latest bash
The above command will create a docker container using the ubuntu image and then run the container and start the bash process of that container so that we can connect and interact with it.
How to Check How Many Docker Containers You Have
docker ps docker ps -la docker ps -a
You can be explicit and add the container command to
docker container ps -a
How to Start A Container
docker start mycontainer_one
How to Stop A Running Container
There are two ways you can stop a running container. These include using the stop or the kill command
docker stop mycontainer_one docker kill mycontainer_one
In case you want to stop and start your container you can use the restart command which in most cases is faster than combining the two commands.
docker restart mycontainer_one
Commands to Connect to a Docker Container
In case you want to connect to a running docker container and execute a command you can use the exec.
docker exec -it mycontainer_one bash docker exec -it mycontainer_one ls
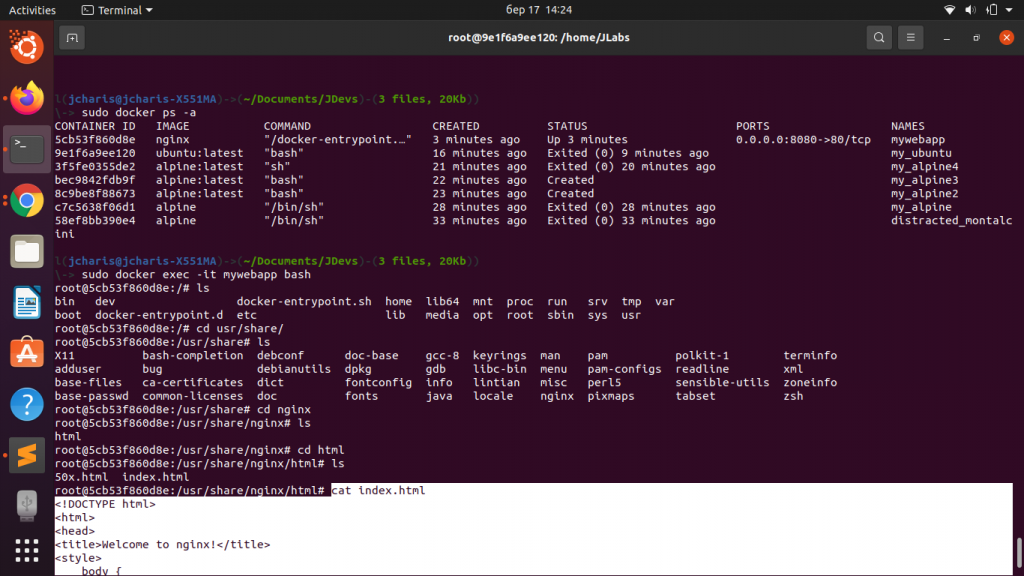
You can also attach to a running container using the attach command
docker attach mycontainer_one
How to Copy Files to Your Docker Container
In case you want to copy files to your docker container from your local system to the docker container you can use the cp command. Let us setup an nginx webserver and modify the index.html file on the webserver.
docker pull nginx
docker run –name mywebapp -p 8080:80 nginx -d bash
We can now open our browser and check the url localhost:8080 to see our nginx web site
docker cp myfile <containerId>:/file/path/within/container
docker cp index.html mywebapp_container:usr/share/nginx/html
How to Copy Files From Your Docker Container to Your Local System
docker cp <containerId>:/file/path/within/container /host/path/target
How to Delete or Remove Docker Images and Docker Containers
docker rm mycontainer_id
To remove an image you can use the rmi command
docker rmi nginx
How to Build Docker Images From A Dockerfile
A Dockerfile is a file that contains layers or steps of instructions that a docker engine or daemon uses to build a docker image and from which we can spin up a docker container instance.. The basic essential commands used on a dockerfile include
The basic set of instructions for any dockerfile include
FROM <image>: this is from where you want to build the base image or operating system.
RUN <command>: this command tells the docker daemon to run a set of commands or programs either via
- shell form : RUN pip install -r requirements.txt
- the exec form: RUN [“pip”,”install”,”-r”,”requirements.txt”]
WORKDIR <directory>: this shows the working directory we will be setting up within our image
ENTRYPOINT [commands] : Allows for configuring the container as an executable.The entrypoint is similar to the CMD in that they serve as a point for what command to run in the container not the image, but rather when you create a container from an image.
CMD <commands>: this is used to provide defaults for an executing container. You can use the shell form or the exec form . When you use both together it is recommended to use it in the exec form that follows the JSON array format.
EXPOSE <port>: This informs Docker that the container is exposed to listen on the specified port at runtime.
COPY <source> <destination> :For copying files from one place to the image.
An example of a docker file is as below
FROM python:3.7 COPY . /app WORKDIR /app RUN pip install streamlit EXPOSE 8501 ENTRYPOINT ["streamlit","run"] CMD ["app.py"]
In order to build the image you can use the build command
To build an image you can run the following command
docker build -t <imagename and tagname> .
or with the -f option if you don’t want to look for the dockerfile in the current directory or context.
(This is useful for when you have one dockerfile for production and another for development)
docker build -t <imagename and tagname> -f Dockerfile
To conclude we have seen some of the most used docker commands. Of course there are several commands but these are some of the most used. If you have any commonly used docker command you can let us know in the comment section below.
Thanks for your time
Jesus Saves
By Jesse E.Agbe(JCharis)