QR-code s a type of matrix barcode mostly 2-dimenstional barcode that was invented in 1994 by Denso Wave a Japanese automotive company. It stands for Qucik Response code.
In this tutorial we will explore how to generate our own QR-code using python and also how to decode the generated QR-code.
By the end of this tutorial you will know
- How to Generate QR-Code
- How to save QR-code as images
- How to change the background color of QR Code
- How to decode a QR-Code
Let us begin
Installation
Python offers a wonderful package called qrcode that allows us to generate qr-code. This package is already available on PyPI so you can install it with pip as below
pip install qrcode
# Alternatively with PILLOW support
pip install qrcode[pil]
Generating QR-Code
To generate a QR-code for any data be it text, urls or numbers we can use two main methods – the API option or using the CLI version of the package.
For the API version we can use the simple method or the more advanced method as below.
import qrcode
# Method 1: Simple Method
img = qrcode.make("this is some awesome package")
img.save("myfirst_file.png")
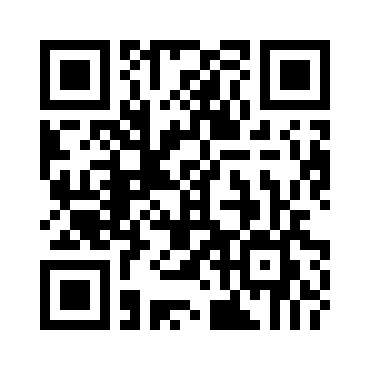
For the more advanced method you can use the class object and create an instance in order to generate your qr-code.
# Create an Instance
qr = qrcode.QRCode(version=1,
error_correction=qrcode.constants.ERROR_CORRECT_M,
box_size=10, border=4,)
# Add Data
qr.add_data("https://jcharistech.com")
qr.make(fit=True)
# Save QR-code as image
img2 = qr.make_image(fill_color='black',back_color='white')
img2.save("mysecond_file.png")
With this package you can generate qr-code. In case you want to change the fill_color or the back_color, you can do so respectively eg.
img2 = qr.make_image(fill_color='blue',back_color='white')
img2.save("mysecond_file2.png")
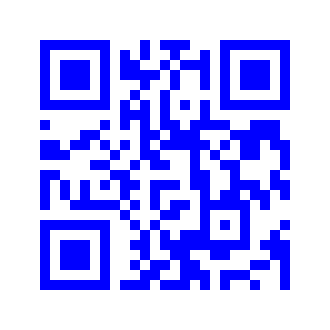
For the CLI option you can launch qrcode from your terminal and then specify your data as below
Now let us move on to the next step, how to decode our generated qr-code.
Decoding QR-Code In Python
In order to decode the generated qr-code image, we will use opencv. Opencv is a powerful computer vision library useful for doing computer vision task as well as image manipulation. To install it you can use pip as below
pip install python-opencv
# How to Decode
import cv2
detector= cv2.QRCodeDetector()
reval,point,s_qr = detector.detectAndDecode(cv2.imread('myfirst_file.png'))
# Decoded Text or Data
print(reval)
# Numpy Array/Matrix of Points
print("Points")
print(point)
# Straight QR
print(s_qr)
To conclude we have seen how to generate QR-code using python. You can also check out the video tutorial below.