Pygwalker is a python framework with a look and feel like Tableau, that allows data scientists to analyze data and visualize patterns with simple drag-and-drop operations. In this tutorial we will see how to use PyGWalker in Flask. We will build a web application whereby a user can upload a csv file and analyze it using pygwalker.
Let us start
Installation of Packages
The packages for this application involves flask, pygwalker, pandas and flask-reuploaded.
pip install flask pandas pygwalker Flask-Reuploaded
Basic Idea
Our app has two parts,
- the frontend from which we will upload our csv file and render our result
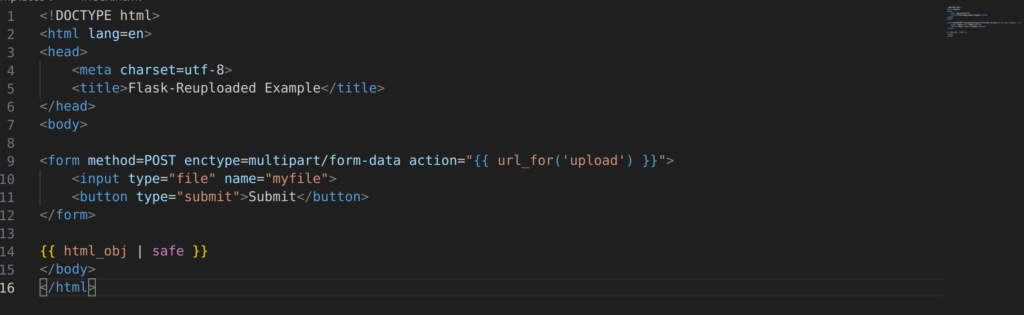
- the backend from which we will process our uploaded files
The basic idea behind the app is that after uploading a csv file we will read it with pandas and then process it with pygwalker, after which we will convert it to html and render it in the frontend via jinja.
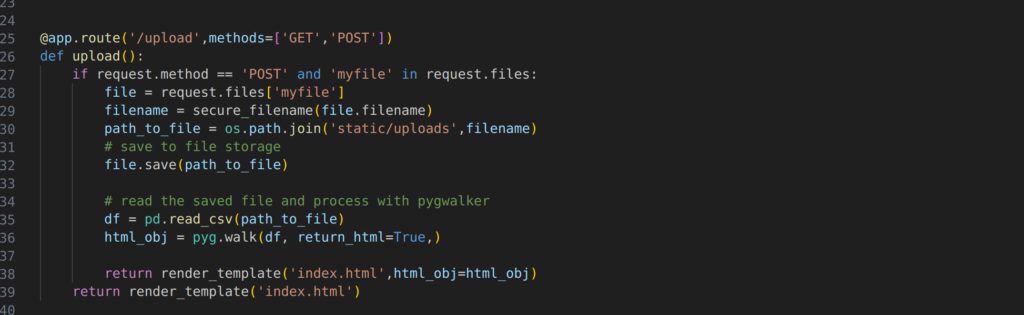
When working with pygwalker, the basic example is
import pygwalker as pyg import pandas as pd # read data df = pd.read_csv("data.csv") # Render with Pygwalker pyg.walk(df) # Get Pygwalker as html html_str = pyg.walk(df,return_html=True)
With the `return_html=True` we can pass this into Jinja Templating syntax and render it as html again via the safe filter as below
{{ html_str | safe }}
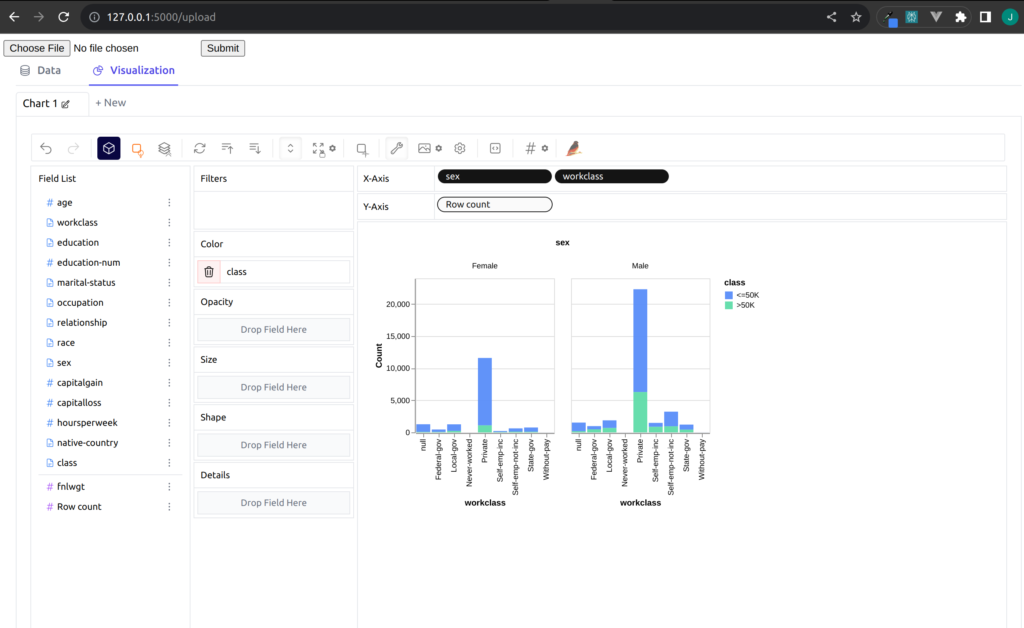
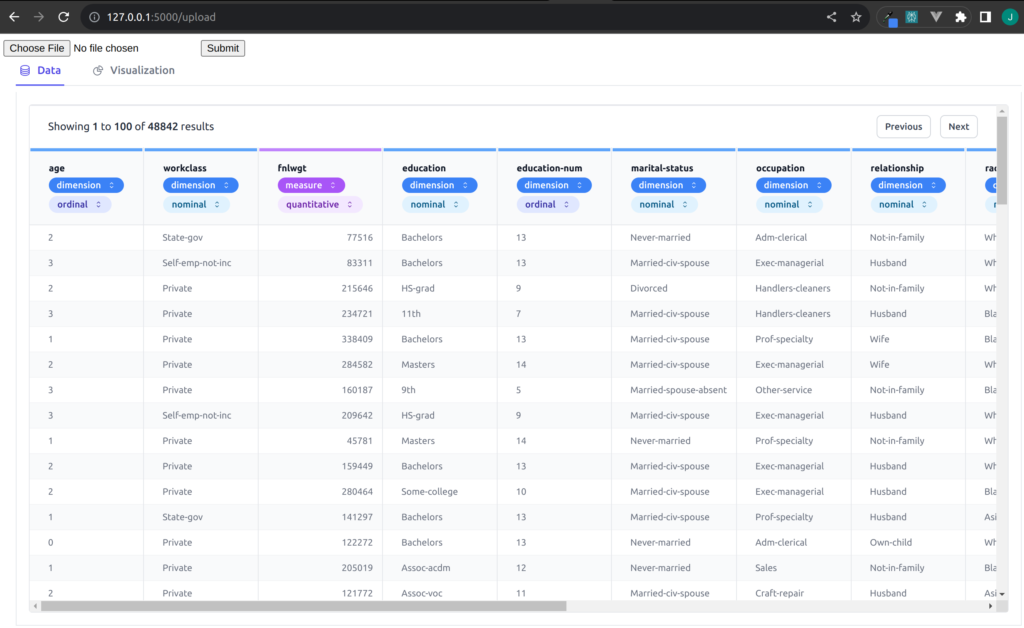
Below is the full code for both the file upload and the rendering in the frontend
from flask import Flask, request,render_template from flask_uploads import UploadSet,configure_uploads,ALL,DATA import os from werkzeug.utils import secure_filename import pygwalker as pyg import pandas as pd app = Flask(__name__) # Configuration for File Uploads files = UploadSet('files',ALL) app.config['UPLOADED_FILES_DEST'] = 'static/uploads' #app.config['MAX_CONTENT_LENGTH'] = 1024 * 10000 app.config['UPLOAD_EXTENSIONS'] = ['.csv', '.txt'] configure_uploads(app,files) @app.route('/') def index(): return render_template('index.html') @app.route('/upload',methods=['GET','POST']) def upload(): if request.method == 'POST' and 'myfile' in request.files: file = request.files['myfile'] filename = secure_filename(file.filename) path_to_file = os.path.join('static/uploads',filename) # save to file storage file.save(path_to_file) # read the saved file and process with pygwalker df = pd.read_csv(path_to_file) html_obj = pyg.walk(df, return_html=True,) return render_template('index.html',html_obj=html_obj) return render_template('index.html') if __name__ == '__main__': app.run(debug=True)
We have seen how to build a simple application using flask and pygwalker, the same idea can be applied for streamlit, django, etc. You can also check out the video tutorial below
Thank you for your attention
Jesus Saves
By Jesse E.Agbe(JCharis)