In this tutorial we will explore a two methods for creating a table where by
we can click on each row and copy the data to clipboard respectively.
This is quite useful when you want to add copy row data instead of the entire table.
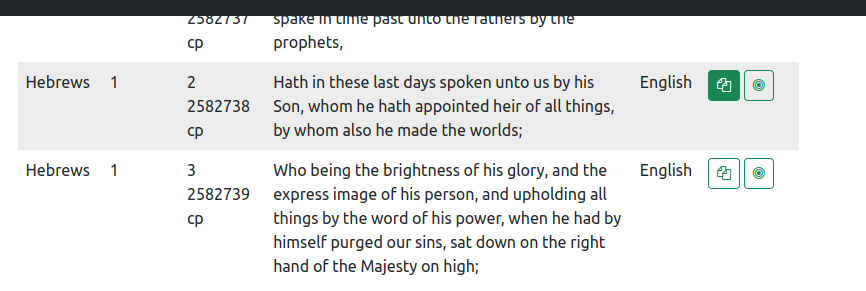
Let us see how to do that.
Designing the Table
We will be fetching our data from a django model and looping through it on the frontend (html) using jinja templating as below
In order to select a row we will pass the current id of our data in the id
element tag of our table or input tag.
<table class="table table-hover table-borderless">
<thead>
<tr>
<th scope="col">Book Name</th>
<th scope="col">title</th>
<th scope="col">Text</th>
<th scope="col">Action</th>
</tr>
</thead>
{% for item in books_list %}
<tbody>
<tr>
<td>{{ item.book_name }}</td>
<td>{{ item.title }}</td>
<td id="{{ item.id |stringformat:'s cp'}}"> {{ item.text }}</td>
<!-- <td><input type="text" value="{{ item.text }}" id="{{ item.id |stringformat:'s cp'}}"></td>-->
<td><button class="btn btn-outline-success btn-sm" onclick="CopyText2({{ item.id}})" data-toggle="tooltip" data-placement="top" title="Copy"><i class="fa fa-copy"></i></button>
</td>
</tr>
</tbody>
{% endfor %}
</table>
Building the Javascript Copy To Clipboard Function
There are several way we can accomplish this ,however we will pick these two.
The first method will be using input
tag and selecting the value when the button is clicked. This approach is good if you want your users to be able to edit the data.
<tbody>
<tr>
<td>{{ item.book_name }}</td>
<td>{{ item.title }}</td>
<td><input type="text" value="{{ item.text }}" id="{{ item.id}}"></td>
<td><button class="btn btn-outline-success btn-sm" onclick="CopyText({{ item.id}})" data-toggle="tooltip" data-placement="top" title="Copy"><i class="fa fa-copy"></i></button>
</td>
</tr>
</tbody>

Add this to your scripts
{% block scripts %}
<script>
// Copy to ClipBoard
function CopyText(el) {
var copyText = document.getElementById(el)
console.log(copyText)
// Select the text field
copyText.select();
copyText.setSelectionRange(0, 99999); // For mobile devices
// Copy the text inside the text field
navigator.clipboard.writeText(copyText.value);
};
</script>
{% endblock scripts %}
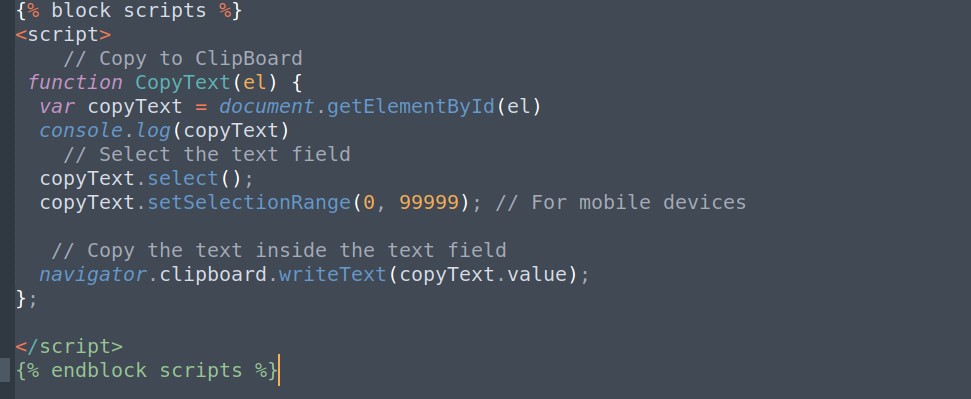
The second method will be using the innerHTML
option which will allow us to copy every data inside a div or a tag. This approach is good if you want to prevent your users from editing the original data.
<tbody>
<tr>
<td>{{ item.book_name }}</td>
<td>{{ item.title }}</td>
<td id="{{ item.id |stringformat:'s cp'}}"> {{ item.text }}</td>
<td><button class="btn btn-outline-success btn-sm" onclick="CopyText({{ item.id}})" data-toggle="tooltip" data-placement="top" title="Copy"><i class="fa fa-copy"></i></button>
</td>
</tr>
</tbody>
{% block scripts %}
<script>
// Copy to ClipBoard
function CopyText2(el) {
// Get the Selected Data By ID
var copyText = document.getElementById(`${el}`)
var str = copyText.innerHTML // Get All HTML Data and Copy to Clipboard
function listener(e) {
e.clipboardData.setData("text/html", str);
e.clipboardData.setData("text/plain", str);
e.preventDefault();
}
document.addEventListener("copy", listener);
document.execCommand("copy");
document.removeEventListener("copy", listener);
};
</script>
{% endblock scripts %}
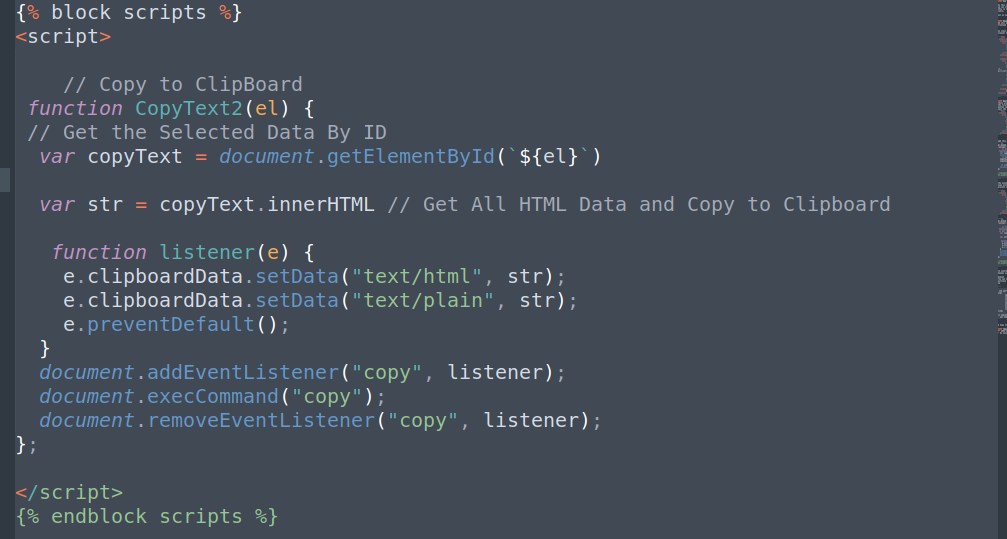
To conclude we have seen how to add a copy to clipboard for our tables in Django via click of a button in Javascript.
Bonus:
You can concat an id and a string to fix issues of having the same id when using
multiple action button on a table row.
eg
<td id="{{ item.id |stringformat:'s dupl'}}"> {{ item.text }}</td>
<td>
<button class="btn btn-outline-success btn-sm" onclick="CopyText({{ item.id}})" data-toggle="tooltip" data-placement="top" title="Copy"><i class="fa fa-copy"></i>
</button>
<button class="btn btn-outline-success btn-sm" onclick="ToggleText({{ item.id }})" data-toggle="tooltip" data-placement="top" title="View"><i class="fa fa-bullseye"></i>
</button>
</td>
// concat the id to our string `${el} dupl` or el + ' dupl'
var copyText = document.getElementById(`${el} dupl`)
I hope this was useful.