Object Oriented Programming is a vital feature when it comes to writing code. In Python, everything can be seen as an object and one of the ways of creating objects in python is via ‘Class’.
A Class is like an object constructor, or a “blueprint” for creating objects.
When working with classes one of the errors you may face is the
`TypeError: ‘X’ object is not subscriptable ‘ where X is the name of your class.
How do you fix this error?
First of all the reason for this error is because
- Treating the object as if it is a dictionary instead of an object.
Lets go into details.
Let make a class called User that has some attributes and no method.
class User:
...: def __init__(self,id=None,name=None,age=None):
...: self.id = id
...: self.name = name
...: self.age = age
...: def __repr__(self):
...: return 'User(id={},name={},age={})'.format(self.id,self.name,self.age)
We can then create an object and check out the first attribute to simulate our type error.
>>> u = User(id=1,name='Jesse',age=25)
>>> # This will work
>>> u.name
>>> 'Jesse'
>>> # This will fail
>>> u['name']
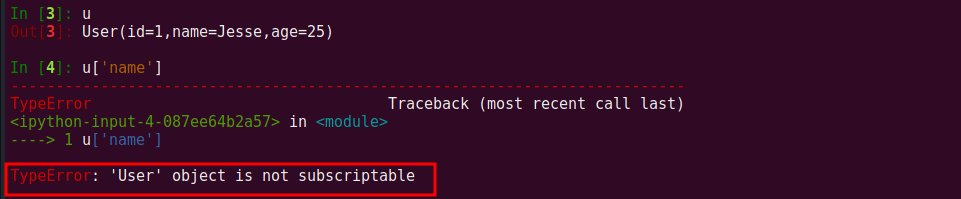
As you can see treating the object like a dictionary would result in this object not subscriptable error ie u[‘name’] or bracket notation syntax.
However if you treat it as an object with attribute no error occurs i.e u.name or the dot syntax.
So in order to fix it you must treat it as an object with attribute and use the dot syntax instead of the bracket syntax.
In case you want to use both the dot syntax and bracket syntax, you will have to add the __getitem__
method to the class as below
class User:
...: def __init__(self,id=None,name=None,age=None):
...: self.id = id
...: self.name = name
...: self.age = age
...: def __repr__(self):
...: return 'User(id={},name={},age={})'.format(self.id,self.name,self.age)
...: def __getitem__(self, key):
...: return self.__dict__[key]
By adding the __getitem__
you can use both methods.
This will fix the issue.
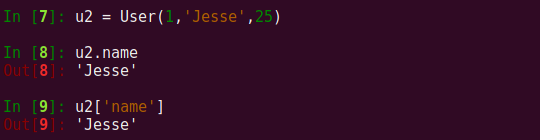
I hope this helps.
Thanks For Your Time
Jesus Saves @JCharisTech
By Jesse E.Agbe(JCharis)